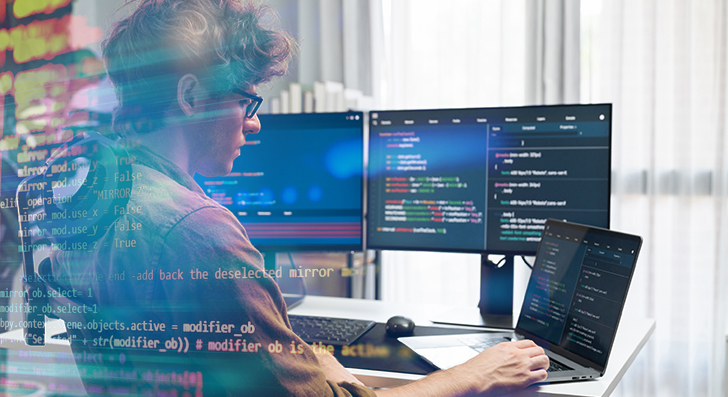
Scalability signifies your software can tackle advancement—far more consumers, more details, plus more website traffic—devoid of breaking. Like a developer, developing with scalability in your mind will save time and anxiety later. Below’s a clear and realistic guidebook that will help you get started by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability is just not anything you bolt on later—it ought to be part of your respective strategy from the start. Numerous apps fall short when they increase quick mainly because the original layout can’t handle the additional load. To be a developer, you should Believe early regarding how your system will behave under pressure.
Get started by developing your architecture being flexible. Keep away from monolithic codebases where by all the things is tightly connected. Alternatively, use modular structure or microservices. These patterns split your application into smaller, independent areas. Each individual module or assistance can scale By itself with out impacting The full procedure.
Also, consider your database from day just one. Will it have to have to manage 1,000,000 buyers or just a hundred? Choose the appropriate form—relational or NoSQL—dependant on how your data will develop. System for sharding, indexing, and backups early, Even when you don’t need them however.
Yet another crucial position is to stop hardcoding assumptions. Don’t produce code that only will work less than existing problems. Contemplate what would transpire If the person base doubled tomorrow. Would your app crash? Would the database slow down?
Use design and style designs that assist scaling, like concept queues or celebration-pushed programs. These enable your application take care of a lot more requests with no receiving overloaded.
Any time you Establish with scalability in your mind, you are not just planning for achievement—you are lowering long run problems. A very well-prepared technique is simpler to keep up, adapt, and increase. It’s far better to organize early than to rebuild later.
Use the correct Database
Choosing the ideal databases is often a essential Portion of creating scalable applications. Not all databases are crafted a similar, and utilizing the Erroneous one can slow you down or simply bring about failures as your app grows.
Start by being familiar with your knowledge. Is it extremely structured, like rows in a desk? If Sure, a relational databases like PostgreSQL or MySQL is an efficient match. These are solid with relationships, transactions, and regularity. Additionally they help scaling procedures like read through replicas, indexing, and partitioning to handle additional site visitors and data.
When your data is much more adaptable—like consumer activity logs, products catalogs, or paperwork—take into consideration a NoSQL alternative like MongoDB, Cassandra, or DynamoDB. NoSQL databases are improved at handling substantial volumes of unstructured or semi-structured info and will scale horizontally a lot more quickly.
Also, look at your study and produce patterns. Have you been accomplishing plenty of reads with less writes? Use caching and skim replicas. Are you currently dealing with a significant write load? Take a look at databases that will cope with superior create throughput, as well as celebration-primarily based info storage devices like Apache Kafka (for temporary info streams).
It’s also good to think forward. You may not will need Highly developed scaling features now, but selecting a database that supports them signifies you gained’t will need to modify later.
Use indexing to speed up queries. Stay away from unneeded joins. Normalize or denormalize your facts based upon your obtain styles. And always keep track of database overall performance while you improve.
To put it briefly, the ideal databases relies on your application’s composition, velocity desires, And exactly how you be expecting it to improve. Acquire time to pick sensibly—it’ll help you save loads of hassle afterwards.
Enhance Code and Queries
Rapidly code is vital to scalability. As your app grows, each small hold off provides up. Improperly published code or unoptimized queries can decelerate efficiency and overload your technique. That’s why it’s crucial that you Construct effective logic from the beginning.
Start out by producing clear, basic code. Stay away from repeating logic and remove just about anything unwanted. Don’t select the most complicated solution if a straightforward a single functions. Keep the features brief, focused, and straightforward to test. Use profiling tools to uncover bottlenecks—spots exactly where your code usually takes way too lengthy to operate or employs an excessive amount of memory.
Future, evaluate your database queries. These normally sluggish things down a lot more than the code itself. Be sure Every question only asks for the data you really have to have. Keep away from Pick *, which fetches everything, and alternatively choose precise fields. Use indexes to speed up lookups. And stay away from accomplishing too many joins, In particular throughout huge tables.
For those who discover the exact same info remaining requested time and again, use caching. Shop the effects temporarily working with tools like Redis or Memcached and that means you don’t have to repeat pricey functions.
Also, batch here your databases functions whenever you can. In lieu of updating a row one by one, update them in groups. This cuts down on overhead and can make your application much more productive.
Make sure to exam with huge datasets. Code and queries that get the job done fine with 100 documents might crash once they have to deal with 1 million.
Briefly, scalable applications are rapid applications. Keep the code limited, your queries lean, and use caching when needed. These steps assist your application keep clean and responsive, at the same time as the load boosts.
Leverage Load Balancing and Caching
As your application grows, it's got to take care of extra buyers and more traffic. If every thing goes via 1 server, it'll rapidly turn into a bottleneck. That’s the place load balancing and caching can be found in. These two resources assist keep your application rapid, steady, and scalable.
Load balancing spreads incoming targeted traffic across numerous servers. Rather than one server doing all of the perform, the load balancer routes customers to various servers based on availability. This suggests no one server will get overloaded. If a single server goes down, the load balancer can send visitors to the Some others. Equipment like Nginx, HAProxy, or cloud-primarily based options from AWS and Google Cloud make this straightforward to set up.
Caching is about storing details briefly so it can be reused immediately. When end users request the same facts once again—like a product site or even a profile—you don’t need to fetch it with the database when. You can provide it from the cache.
There's two typical different types of caching:
1. Server-facet caching (like Redis or Memcached) merchants data in memory for rapid access.
two. Client-aspect caching (like browser caching or CDN caching) stores static documents close to the person.
Caching minimizes databases load, improves pace, and makes your app extra effective.
Use caching for things which don’t alter generally. And usually ensure that your cache is updated when knowledge does change.
In short, load balancing and caching are basic but powerful resources. Jointly, they help your app take care of more consumers, keep fast, and Recuperate from challenges. If you propose to develop, you require both.
Use Cloud and Container Instruments
To build scalable applications, you may need instruments that permit your app develop simply. That’s wherever cloud platforms and containers are available. They give you flexibility, minimize setup time, and make scaling Considerably smoother.
Cloud platforms like Amazon World-wide-web Services (AWS), Google Cloud Platform (GCP), and Microsoft Azure Enable you to lease servers and expert services as you would like them. You don’t have to purchase hardware or guess potential ability. When targeted traffic improves, you could increase more resources with just a few clicks or immediately utilizing car-scaling. When website traffic drops, you may scale down to economize.
These platforms also present expert services like managed databases, storage, load balancing, and stability applications. You may center on setting up your application as an alternative to controlling infrastructure.
Containers are One more crucial Device. A container deals your app and everything it must run—code, libraries, configurations—into one particular unit. This makes it quick to maneuver your app between environments, from a laptop computer for the cloud, with out surprises. Docker is the most popular Resource for this.
Whenever your app takes advantage of a number of containers, equipment like Kubernetes make it easier to deal with them. Kubernetes handles deployment, scaling, and recovery. If a person aspect of the app crashes, it restarts it mechanically.
Containers also ensure it is easy to individual elements of your application into companies. You are able to update or scale sections independently, which can be perfect for functionality and reliability.
Briefly, utilizing cloud and container resources usually means you'll be able to scale fast, deploy simply, and recover speedily when problems come about. If you want your app to mature without having restrictions, begin working with these tools early. They preserve time, cut down danger, and make it easier to stay centered on building, not repairing.
Watch Everything
In case you don’t watch your application, you won’t know when factors go Completely wrong. Monitoring aids the thing is how your application is accomplishing, spot concerns early, and make superior conclusions as your app grows. It’s a important Section of making scalable techniques.
Start off by monitoring essential metrics like CPU usage, memory, disk Area, and response time. These inform you how your servers and expert services are accomplishing. Tools like Prometheus, Grafana, Datadog, or New Relic will help you acquire and visualize this knowledge.
Don’t just watch your servers—observe your application too. Keep an eye on how long it takes for customers to load webpages, how often mistakes transpire, and wherever they come about. Logging applications like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will let you see what’s going on within your code.
Arrange alerts for vital problems. For example, In case your response time goes above a limit or a service goes down, you need to get notified immediately. This helps you take care of challenges speedy, normally in advance of people even notice.
Checking is likewise handy after you make improvements. When you deploy a whole new attribute and see a spike in faults or slowdowns, you may roll it back again prior to it leads to serious problems.
As your app grows, traffic and facts enhance. With out checking, you’ll overlook signs of issues until finally it’s too late. But with the appropriate applications in position, you stay on top of things.
In short, checking assists you keep the app responsible and scalable. It’s not nearly recognizing failures—it’s about knowing your system and making certain it really works properly, even stressed.
Ultimate Views
Scalability isn’t just for significant organizations. Even compact apps will need a strong Basis. By designing meticulously, optimizing sensibly, and utilizing the right equipment, you can Construct applications that develop efficiently without breaking under pressure. Start out small, Feel significant, and Develop sensible.